Request failed for https://gql.waveapps.com returned code 500.
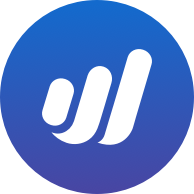
The following script runs fine on my local browser. However, when I tried to run it through Google Apps Script, I received the following error.
Exception: Request failed for https://gql.waveapps.com returned code 500. Truncated server response: POST body missing. Did you forget use body-parser middleware? (use muteHttpExceptions option to examine full response). (line 5, file "Upload Invoices")
and here's the script I used;
function queryFetch(query, variables) {
var url = ('https://gql.waveapps.com/graphql/public');
return UrlFetchApp.fetch(url, {
method: 'POST',
headers: {
"Authorization": "Bearer ",
'Content-Type': 'application/json',
},
body: JSON.stringify({
query: query,
variables: variables
})
})
.then(r => r.json());
}
function getInvoice() {
var ui = SpreadsheetApp.getUi();
var invoiceNumber_entry = invoiceNumber_prompt.getResponseText();
queryFetch(query($businessId: ID!, $invoiceNumber: String) { business(id: $businessId) { invoices(invoiceNumber: $invoiceNumber) { edges { node { invoiceNumber invoiceDate customer {name} taxTotal {value} total {value} memo items { product {name} description quantity unitPrice total {value} taxes {amount {value}} } } } } } }
, {
"businessId": "",
"invoiceNumber": invoiceNumber})
.then(data => {
console.log(data);
});
}
Could someone tell me how I can fix this?
Comments
This error is emitted if no post body was used. Do you get any errors emitted when queryFetch is called? It looks like JSON.stringify may be returning an empty string.
HI, thanks for the quick response!
I guess the problem might be that I'm not using the Google apps script's UrlFetchApp Class (https://developers.google.com/apps-script/reference/url-fetch/url-fetch-app#fetch(String,Object)) in the right way (and yes! all of this works perfectly when I execute it from a local server!)...
Looking up some documentation showed that Apps Script does not use the query key but instead includes it within the payload key ( I'm not sure about this ). I tried fiddling around with the code and skipped the queryFetch() function and put it all into one function.
Here's the code I'm working with right now...
function getCustomers() {
var response = UrlFetchApp.fetch("https://gql.waveapps.com/graphql/public", {
muteHttpExceptions: false,
headers: {
"Authorization": "Bearer <>",
},
contentType: "application/json",
method: 'POST',
payload: {
query:
query ($businessId: ID!) { business(id: $businessId) { id customers(sort: [NAME_ASC]) { edges{ node { name id } } } } }
,variables: {"businessId": "<>"}
}
});
Logger.log(response.getContentText())
}
But now I'm getting an error like this;
Request failed for https://gql.waveapps.com returned code 400. Truncated server response: <!DOCTYPE html> Error
(use muteHttpExceptions option to examine full response).
I feel someone who has used google apps script to perform queries with Wave Api earlier could direct me in the right way...
Hi! Thank you for the response...
I was able to resolve the issue... turns out UrlFetchApp class of Google Scripts uses the keyword 'payload' instead of 'body'. On changing that and making some other modifications, I was able to query the details successfuly...
I'm leaving the code here just in case someone else might find it helpful...
function getCustomers() {
var url = 'https://gql.waveapps.com/graphql/public';
var query =
query ($businessId: ID!) { business(id: $businessId) { id customers(sort: [NAME_ASC]) { edges{ node { name id } } } } }
var variables = {
"businessId": ""
};
var params = {
method: 'POST',
headers: {
"Authorization": "Bearer ",
'Content-Type': 'application/json'
},
payload: JSON.stringify({
query: query,
variables: variables
})
};
var response = UrlFetchApp.fetch(url, params);
var data = (response.getContentText());
console.log(data);
}
@thomasmathew this was a life saver, that one little keyword threw me off. Thank you for posting the code, it DID help someone down the road!